With the aid of examples, you will learn about the break statement, also known as the break statement in Java, in this tutorial.
When using loops, it may be desired to skip parts of the loop’s statements or to end the loop right away without running the test expression.
Break and continue statements are employed in certain circumstances. The next tutorial will cover the Java continue statement.
The break statement in Java immediately ends the loop and the program’s control shifts to the statement that follows the loop.
It nearly always goes with declarative decisions (Java if…else Statement).
The Java syntax for the break statement is as follows:
break;
How break statement works?
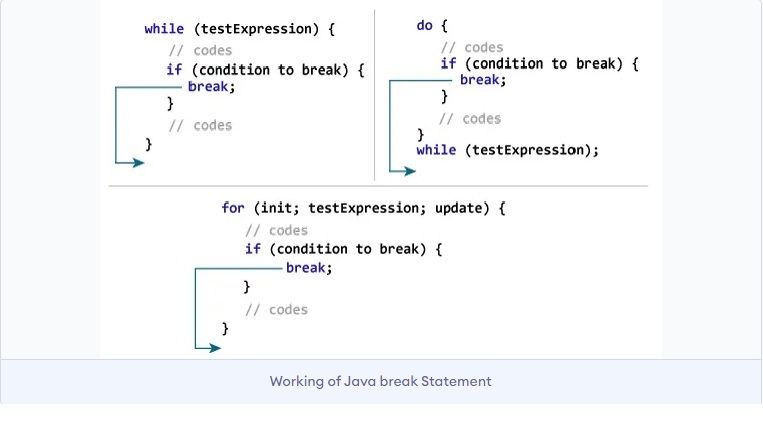
Example 1: Java break statement
class Test {
public static void main(String[] args) {
// for loop
for (int i = 1; i <= 10; ++i) {
// if the value of i is 5 the loop terminates
if (i == 5) {
break;
}
System.out.println(i);
}
}
}
Output
1
2
3
4
The for loop is used in the program above to output the value of I after each iteration. Visit the Java for loop to see how it functions. Take note of this sentence:
if (i == 5) {
break;
}
This indicates that the loop ends when the value of I equals 5. As a result, we only receive the output for values lower than 5.
Example 2: Java break statement
Until the user submits a negative number, the program below calculates the total of the numbers they have entered.
We have utilized the Scanner object to capture user input. Visit Java Scanner to find out more about Scanner.
import java.util.Scanner;
class UserInputSum {
public static void main(String[] args) {
Double number, sum = 0.0;
// create an object of Scanner
Scanner input = new Scanner(System.in);
while (true) {
System.out.print("Enter a number: ");
// takes double input from user
number = input.nextDouble();
// if number is negative the loop terminates
if (number < 0.0) {
break;
}
sum += number;
}
System.out.println("Sum = " + sum);
}
}
Output:
Enter a number: 3.2
Enter a number: 5
Enter a number: 2.3
Enter a number: 0
Enter a number: -4.5
Sum = 10.5
The while loop’s test statement in the program above is always true. Take note of this line:
if (number < 0.0) {
break;
}
This indicates that the while loop is ended when the user inputs negative numbers.
Java break and Nested Loop
The break statement ends the innermost loop when there are nested loops.
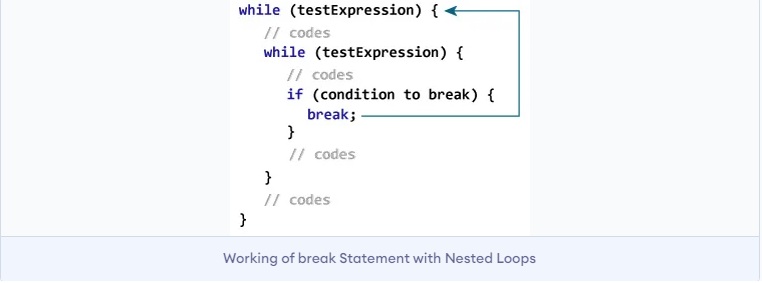
The innermost while loop in this case is terminated by the break statement, and control is transferred to the outer loop.
Labeled break Statement
We have been using the unmarked break statement up to this point. The innermost loop and switch statement are ended. However, Java also supports a different kind of break statement called a named break.
The designated break statement can also be used to end the outermost loop.
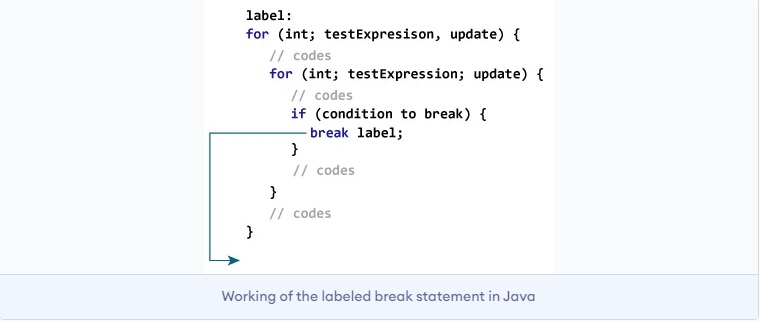
As seen in the graphic above, we have specified the outer loop using the label identifier. Observe how the break statement is applied now (break label;).
In this case, the break statement ends the labeled statement (i.e. outer loop). The program then jumps to the statement after the labeled statement.
Here’s another illustration:
while (testExpression) {
// codes
second:
while (testExpression) {
// codes
while(testExpression) {
// codes
break second;
}
}
// control jumps here
}
In the example above, the while loop designated as second is ended when the statement breaks second; is executed. Following the second while loop, the statement takes control of the program.
Example 3: labeled break Statement
class LabeledBreak {
public static void main(String[] args) {
// the for loop is labeled as first
first:
for( int i = 1; i < 5; i++) {
// the for loop is labeled as second
second:
for(int j = 1; j < 3; j ++ ) {
System.out.println("i = " + i + "; j = " +j);
// the break statement breaks the first for loop
if ( i == 2)
break first;
}
}
}
}
Output:
i = 1; j = 1
i = 1; j = 2
i = 2; j = 1
The labeled break statement is used in the example above to end the loop designated as first. This is,
first:
for(int i = 1; i < 5; i++) {...}
The program will act differently if the statement break first is changed to break second in this case. In this situation, the second loop will come to an end. For instance,
class LabeledBreak {
public static void main(String[] args) {
// the for loop is labeled as first
first:
for( int i = 1; i < 5; i++) {
// the for loop is labeled as second
second:
for(int j = 1; j < 3; j ++ ) {
System.out.println("i = " + i + "; j = " +j);
// the break statement terminates the loop labeled as second
if ( i == 2)
break second;
}
}
}
}
Output:
i = 1; j = 1
i = 1; j = 2
i = 2; j = 1
i = 3; j = 1
i = 3; j = 2
i = 4; j = 1
i = 4; j = 2