With the aid of examples, you will learn about Java’s continue statement and labeled continue statement in this tutorial.
You might occasionally want to skip some statements or end the loop when working with loops. Break and continue statements are employed in certain circumstances.
Visit Java break to learn more about the break statement. We shall study the continued statement in this section.
Java continue
The continue command skips the current loop iteration (for, while, do…while, etc).
The program moves to the end of the loop after the continue command. Additionally, test expression is assessed (update statement is evaluated in case of the for loop).
The continued statement’s syntax is seen below.
continue;
Working of Java continue statement

Example 1: Java continue statement
class Main {
public static void main(String[] args) {
// for loop
for (int i = 1; i <= 10; ++i) {
// if value of i is between 4 and 9
// continue is executed
if (i > 4 && i < 9) {
continue;
}
System.out.println(i);
}
}
}
Outer:
1
2
3
4
9
10
The for loop is used in the program above to output the value of I after each iteration. Visit Java for loop to learn how loops operate. Take note of the sentence,
if (i > 4 && i < 9) {
continue;
}
When the value of I becomes more than 4 and less than 9, the continue statement is executed in this case.
The print statement for those values is subsequently skipped. The output therefore skips the numbers 5, 6, 7, and 8.
Example 2: Compute the sum of 5 positive numbers
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Double number, sum = 0.0;
// create an object of Scanner
Scanner input = new Scanner(System.in);
for (int i = 1; i < 6; ++i) {
System.out.print("Enter number " + i + " : ");
// takes input from the user
number = input.nextDouble();
// if number is negative
// continue statement is executed
if (number <= 0.0) {
continue;
}
sum += number;
}
System.out.println("Sum = " + sum);
input.close();
}
}
Output:
Enter number 1: 2.2
Enter number 2: 5.6
Enter number 3: 0
Enter number 4: -2.4
Enter number 5: -3
Sum = 7.8
The total of five positive values was printed using the for loop in the example above. Observe the line.
if (number < 0.0) {
continue;
}
Here, the continue statement is executed if the user submits a negative value. This moves program control to the loop’s update expression and skips the current iteration of the loop.
Java continues with Nested Loop
The continue statement in a Java nested loop skips the current iteration of the innermost loop.
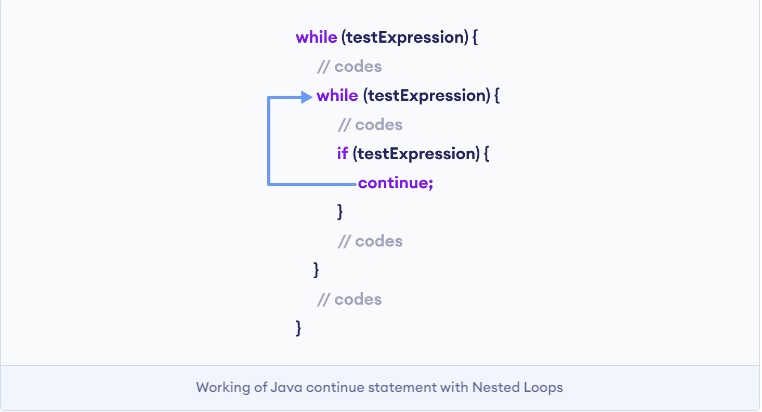
Example 3: continue with Nested Loop
class Main {
public static void main(String[] args) {
int i = 1, j = 1;
// outer loop
while (i <= 3) {
System.out.println("Outer Loop: " + i);
// inner loop
while(j <= 3) {
if(j == 2) {
j++;
continue;
}
System.out.println("Inner Loop: " + j);
j++;
}
i++;
}
}
}
Outer:
Outer Loop: 1
Inner Loop: 1
Inner Loop: 3
Outer Loop: 2
Outer Loop: 3
We used a nested while loop in the example above. Keep in mind that the inner loop contains a continue statement.
if(j == 2) {
j++;
continue:
}
Here, the continue statement is executed and the value of j is raised to 4 when it equals 2.
This omits the inner loop iteration. As a result, the output skips the sentence Inner Loop: 2.
Labeled continue Statement
The unlabeled continue statement has been used up to this point. The labeled continue statement in Java is an additional type of continue statement.
The continue keyword is present, along with the loop’s label. For illustration
continue label;
The continue clause in this case skips the iteration of the loop indicated by the label.

We can see that the outer loop is specified by the label identifier. Keep in mind that the inner loop contains a continue statement.
The labeled statement is being skipped by the continue statement in this case (i.e. outer loop). The next repetition of the labeled statement is then where the program control shifts.
Example 4: labeled continue Statement
class Main {
public static void main(String[] args) {
// outer loop is labeled as first
first:
for (int i = 1; i < 6; ++i) {
// inner loop
for (int j = 1; j < 5; ++j) {
if (i == 3 || j == 2)
// skips the current iteration of outer loop
continue first;
System.out.println("i = " + i + "; j = " + j);
}
}
}
}
Output:
i = 1; j = 1
i = 2; j = 1
i = 4; j = 1
i = 5; j = 1
The labeled continue statement is used in the example above to skip the current iteration of the loop with the label first.
if (i==3 || j==2)
continue first;
Here, we can see that the first loop is the outermost one.
first:
for (int i = 1; i < 6; ++i) {..}
Therefore, if the value of I is 3 or the value of j is 2, the iteration of the outer for loop is skipped.