Through the use of examples and Java’s if and if…else statements, you will learn about control flow statements in this course.
The if..else statement in programming is used to choose amongst several options while running a block of code.
For instance, giving students grades (A, B, or C) based on the percentage they achieved.
- if the percentage is above 90, assign grade A
- if the percentage is above 75, assign grade B
- if the percentage is above 65, assign grade C
1. Java if (if-then) Statement
The syntax of an if-then statement is:
if (condition) {
// statements
}
Condition in this case is a boolean expression, such as age >= 18.
- If the condition is true, the statements are carried out.
- Statements are skipped if the condition evaluates to false.
Working of if Statement
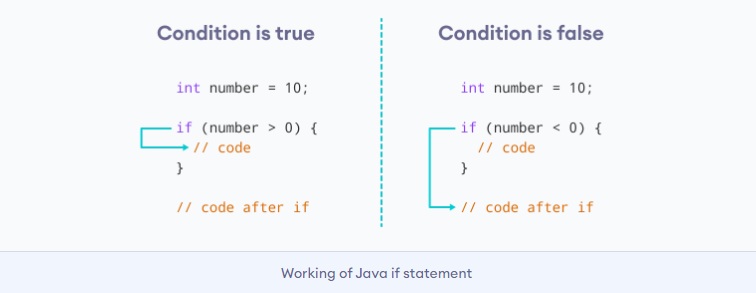
Example 1: Java if Statement
class IfStatement {
public static void main(String[] args) {
int number = 10;
// checks if number is less than 0
if (number < 0) {
System.out.println("The number is negative.");
}
System.out.println("Statement outside if block");
}
}
Output
Statement outside if block
Number 0 is false in the program. As a result, the if statement’s body of code is skipped.
We can also use Java Strings as the test condition.
Example 2: Java if with String
class Main {
public static void main(String[] args) {
// create a string variable
String language = "Java";
// if statement
if (language == "Java") {
System.out.println("Best Programming Language");
}
}
}
Output
Best Programming Language
In the if the block of the example above, we are comparing two strings.
2. Java if…else (if-then-else) Statement
If the test expression evaluates to true, the if statement causes a specific section of code to run. However, nothing happens if the test expression is interpreted as false.
We can employ an optional else block in this situation. If the test expression is assessed as false, the statements included in the else block are carried out. In Java, this is referred to as an if-then statement.
The if…else sentence has the following syntax:
if (condition) {
// codes in if block
}
else {
// codes in else block
}
Here, if the condition is true, the programme will execute the code inside the if block, and if the condition is false, the programme will execute the code inside the else block.
How does the if…else statement work?

Example 3: Java if…else Statement
class Main {
public static void main(String[] args) {
int number = 10;
// checks if number is greater than 0
if (number > 0) {
System.out.println("The number is positive.");
}
// execute this block
// if number is not greater than 0
else {
System.out.println("The number is not positive.");
}
System.out.println("Statement outside if...else block");
}
}
Output
The number is positive.
Statement outside if...else block
We have a variable called the number in the aforementioned example. The test expression number > 0 determines whether the value of the number is greater than 0.
The test statement evaluates to true since the value of the input integer is 10. As a result, the code included in the if’s body is run.
Now make the number’s value a negative integer. Decide on -5.
int number = -5;
The output will be as follows if we run the programme with the new value of the number:
The number is not positive.
Statement outside if...else block
The number here has a value of -5. As a result, the test expression returns a false result. Therefore, the code contained in the else body is run.
3. Java if…else…if Statement
An if…else…if the ladder is available in Java and can be used to execute one block of code while multiple other blocks are being executed.
if (condition1) {
// codes
}
else if(condition2) {
// codes
}
else if (condition3) {
// codes
}
.
.
else {
// codes
}
In this case, if statements are carried out starting at the top and working down. The body of the if block’s code is executed if the test condition is satisfied. And the programme control exits the if…else…if hierarchy.
If every test expression returns false, the body of the else’s function is executed.
How does the if…else…if ladder work?
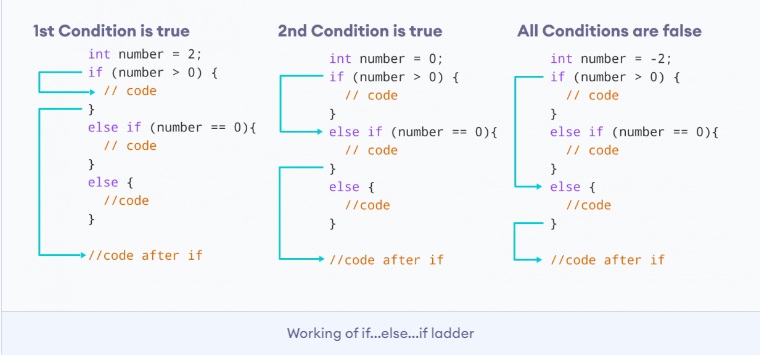
Example 4: Java if…else…if Statement
class Main {
public static void main(String[] args) {
int number = 0;
// checks if number is greater than 0
if (number > 0) {
System.out.println("The number is positive.");
}
// checks if number is less than 0
else if (number < 0) {
System.out.println("The number is negative.");
}
// if both condition is false
else {
System.out.println("The number is 0.");
}
}
}
Output
The Number is 0.
We are determining whether the number in the example above is positive, negative, or zero. We have two condition expressions in this case:
- number > 0 – determines if the number is higher than zero.
- number 0 – determines if the value is less than 0.
The number here has a value of 0. As a result, both criteria are falsely evaluated. Therefore, the statement contained within else’s body is carried out.
4. Java Nested if..else Statement
The usage of if…else statements inside of an if…else statement is also available in Java. The phrase “nested if…else statement” describes it.
Here is a programme that uses nested if…else statements to find the largest of three numbers.
Example 5: Nested if…else Statement
class Main {
public static void main(String[] args) {
// declaring double type variables
Double n1 = -1.0, n2 = 4.5, n3 = -5.3, largest;
// checks if n1 is greater than or equal to n2
if (n1 >= n2) {
// if...else statement inside the if block
// checks if n1 is greater than or equal to n3
if (n1 >= n3) {
largest = n1;
}
else {
largest = n3;
}
} else {
// if..else statement inside else block
// checks if n2 is greater than or equal to n3
if (n2 >= n3) {
largest = n2;
}
else {
largest = n3;
}
}
System.out.println("Largest Number: " + largest);
}
}
output
Largest number: 4.5
To make things easier, we assigned the values of variables ourselves in the scripts mentioned above.
These values, however, might originate from user input information, log files, form submissions, etc. in real-world systems.