A multi-way branch statement is the switch statement. The Java switch statement runs one statement from a variety of criteria, to put it simply. This phrase resembles an if-else-if ladder. It offers a simple method for allocating execution to various sections of code according to the expression’s value. Primitive data types including bytes, shorts, chars, and ints can all be used in the expression. In essence, it checks whether variables are equivalent to a range of values.
Some Important Rules for Switch Statements
- There can be as many cases as there are condition checks, but keep in mind that duplicate case/s values are not permitted.
2. A case’s value has to be of the same data type as the switch’s variable.
3. A case’s value must be a constant or a literal. There is no room for variables.
4. To end a series of statements, the break statement is used inside the switch.
5. The break statement is not required. Execution will move on to the following case if it is left out.
6. The default statement can be inserted anywhere in the switch block and is optional. Keeping a break statement after the default statement will prevent the execution of the next statement if it is not at the end.
Flow Diagram of Switch-Case Statement
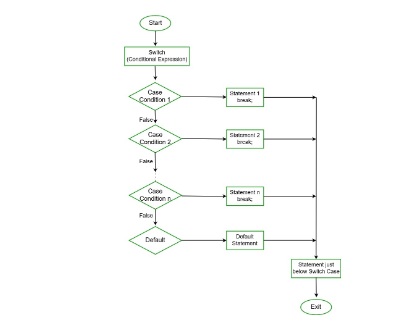
Syntax: Switch-case
// switch statement
switch(expression)
{
// case statements
// values must be of same type of expression
case value1 :
// Statements
break; // break is optional
case value2 :
// Statements
break; // break is optional
// We can have any number of case statements
// below is default statement, used when none of the cases is true.
// No break is needed in the default case.
default :
// Statements
}
Example:
Consider the Java program below, which declares an int with the name day whose value corresponds to a day (1-7). The switch statement in the code is used to display the name of the day dependent on the value of the day.
// Java program to Demonstrate Switch Case
// with Primitive(int) Data Type
// Class
public class GFG {
// Main driver method
public static void main(String[] args)
{
int day = 5;
String dayString;
// Switch statement with int data type
switch (day) {
// Case
case 1:
dayString = "Monday";
break;
// Case
case 2:
dayString = "Tuesday";
break;
// Case
case 3:
dayString = "Wednesday";
break;
// Case
case 4:
dayString = "Thursday";
break;
// Case
case 5:
dayString = "Friday";
break;
// Case
case 6:
dayString = "Saturday";
break;
// Case
case 7:
dayString = "Sunday";
break;
// Default case
default:
dayString = "Invalid day";
}
System.out.println(dayString);
}
}
Output
Friday
Omitting the break Statement
Break statements are not required. Without the break, execution will carry on to the following case. Multiple instances with no break statements in between them are occasionally desirable. Consider the revised version of the aforementioned application, which further indicates whether a day is a weekday or a weekend day.
Example:
// Java Program to Demonstrate Switch Case
// with Multiple Cases Without Break Statements
// Class
public class GFG {
// main driver method
public static void main(String[] args)
{
int day = 2;
String dayType;
String dayString;
// Switch case
switch (day) {
// Case
case 1:
dayString = "Monday";
break;
// Case
case 2:
dayString = "Tuesday";
break;
// Case
case 3:
dayString = "Wednesday";
break;
case 4:
dayString = "Thursday";
break;
case 5:
dayString = "Friday";
break;
case 6:
dayString = "Saturday";
break;
case 7:
dayString = "Sunday";
break;
default:
dayString = "Invalid day";
}
switch (day) {
// Multiple cases without break statements
case 1:
case 2:
case 3:
case 4:
case 5:
dayType = "Weekday";
break;
case 6:
case 7:
dayType = "Weekend";
break;
default:
dayType = "Invalid daytype";
}
System.out.println(dayString + " is a " + dayType);
}
}
Output
Tuesday is weekday
Nested Switch Case statements
A switch can be used as a component of the statement chain of an outer switch. The switch in question is nested. There are no conflicts between the case constants in the inner switch and those in the outer switch because a switch statement creates its own block.
Example:
// Java Program to Demonstrate
// Nested Switch Case Statement
// Class
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Custom input string
String Branch = "CSE";
int year = 2;
// Switch case
switch (year) {
// Case
case 1:
System.out.println(
"elective courses : Advance english, Algebra");
// Break statement to hault execution here
// itself if case is matched
break;
// Case
case 2:
// Switch inside a switch
// Nested Switch
switch (Branch) {
// Nested case
case "CSE":
case "CCE":
System.out.println(
"elective courses : Machine Learning, Big Data");
break;
// Case
case "ECE":
System.out.println(
"elective courses : Antenna Engineering");
break;
// default case
// It will execute if above cases does not
// execute
default:
// Print statement
System.out.println(
"Elective courses : Optimization");
}
}
}
}
Output
elective courses : Machine Learning, Big Data
Example:
// Java Program to Illustrate Use of Enum
// in Switch Statement
// Class
public class GFG {
// Enum
public enum Day { Sun, Mon, Tue, Wed, Thu, Fri, Sat }
// Main driver method
public static void main(String args[])
{
// Enum
Day[] DayNow = Day.values();
// Iterating using for each loop
for (Day Now : DayNow) {
// Switch case
switch (Now) {
// Case 1
case Sun:
System.out.println("Sunday");
// break statement that hault further
// execution once case is satisfied
break;
// Case 2
case Mon:
System.out.println("Monday");
break;
// Case 3
case Tue:
System.out.println("Tuesday");
break;
// Case 4
case Wed:
System.out.println("Wednesday");
break;
// Case 5
case Thu:
System.out.println("Thursday");
break;
// Case 6
case Fri:
System.out.println("Friday");
break;
// Case 7
case Sat:
System.out.println("Saturday");
break;
}
}
}
}
Output
Sunday
Monday
Tuesday
Wednesday
Thursday
Friday
Saturday