With the aid of examples, we will learn how to use the while and do while loops in Java in this article.
Loops are used in computer programming to repeatedly run a block of code. A loop can be used, for instance, to repeat a message 100 times. It’s only a simple illustration; loops may be used to accomplish much more.
You studied the Java for loop in the prior lesson. You will discover more about while and do…while loops in this article.
Java while loop
While loops in Java are used to execute a piece of code until a specified condition is met. The while loop’s syntax is as follows:
while (testExpression) {
// body of loop
}
Here,
- The text expression inside the parenthesis is evaluated by a while loop ().
2. The while loop’s code is run if the test expression evaluates to true.
3. Expression is assessed once again.
4. Until the text Expression is false, this process keeps on.
5. The loop ends when the test expression evaluates to false.
Flowchart of while loop
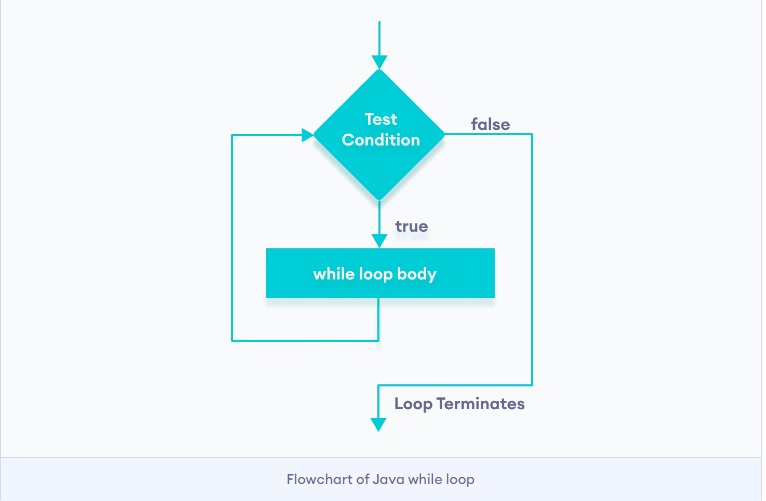
Example 1: Display Numbers from 1 to 5
// Program to display numbers from 1 to 5
class Main {
public static void main(String[] args) {
// declare variables
int i = 1, n = 5;
// while loop from 1 to 5
while(i <= n) {
System.out.println(i);
i++;
}
}
}
Output
1
2
3
4
5
Here is how this program works.
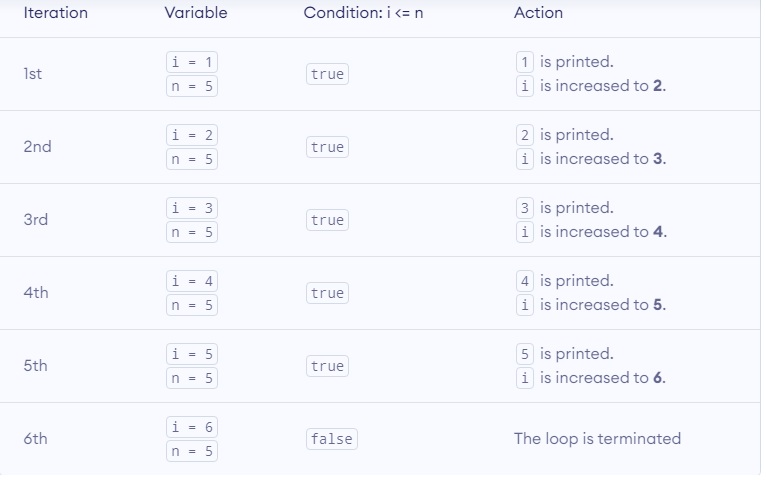
Example 2: Sum of Positive Numbers Only
// Java program to find the sum of positive numbers
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int sum = 0;
// create an object of Scanner class
Scanner input = new Scanner(System.in);
// take integer input from the user
System.out.println("Enter a number");
int number = input.nextInt();
// while loop continues
// until entered number is positive
while (number >= 0) {
// add only positive numbers
sum += number;
System.out.println("Enter a number");
number = input.nextInt();
}
System.out.println("Sum = " + sum);
input.close();
}
}
Output
Enter a number
25
Enter a number
9
Enter a number
5
Enter a number
-3
Sum = 39
The Scanner class was utilised in the aforementioned software to collect user input. NextInt() here accepts user-supplied integer input.
Until the user enters a negative value, the while loop runs continuously. The user-inputted integer is added to the sum variable at the beginning of each iteration.
The loop ends when the user enters a negative number. The final step is to display the total.
Java do…while loop
While loop and do…while loop are related. Before the test expression is tested, however, the do…while loop’s body is run once. For instance,
do {
// body of loop
} while(textExpression);
- The loop’s body is first carried out. The text Expression is then assessed.
2. The body of the loop inside the do statement is repeated execution if the textExpression evaluates to true.
3. The textExpression is once more assessed.
4. The body of the loop inside the do statement is repeated execution if the textExpression evaluates to true.
5. Until the textExpression evaluates to false, this procedure keeps going. The loop then ends.
Flowchart of do…while loop

Let’s see the working of do...while
loop.
Example 3: Display Numbers from 1 to 5
// Java Program to display numbers from 1 to 5
import java.util.Scanner;
// Program to find the sum of natural numbers from 1 to 100.
class Main {
public static void main(String[] args) {
int i = 1, n = 5;
// do...while loop from 1 to 5
do {
System.out.println(i);
i++;
} while(i <= n);
}
}
Output
1
2
3
4
5
Here is how this program works.
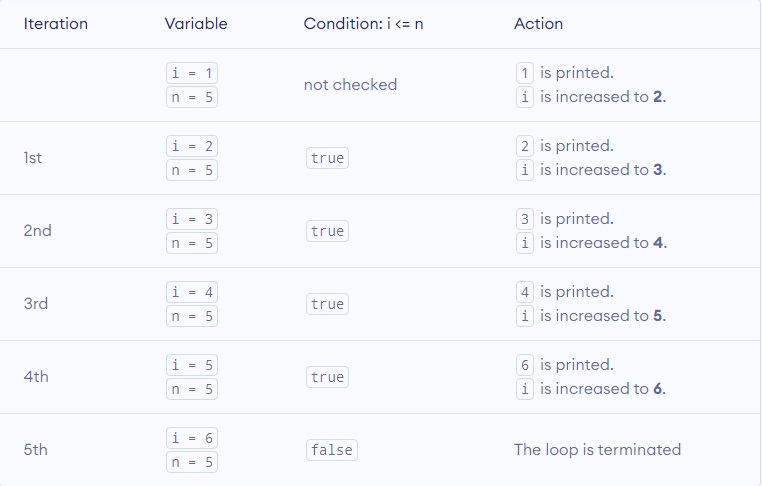
Example 4: Sum of Positive Numbers
// Java program to find the sum of positive numbers
import java.util.Scanner;
class Main {
public static void main(String[] args) {
int sum = 0;
int number = 0;
// create an object of Scanner class
Scanner input = new Scanner(System.in);
// do...while loop continues
// until entered number is positive
do {
// add only positive numbers
sum += number;
System.out.println("Enter a number");
number = input.nextInt();
} while(number >= 0);
System.out.println("Sum = " + sum);
input.close();
}
}
Output 1
Enter a number
25
Enter a number
9
Enter a number
5
Enter a number
-3
Sum = 39
When a user provides a positive value in this case, the sum variable is increased by that amount. And so on, until the number is negative, this process continues. When the value is negative, the loop ends and the sum is shown without the negative value.
Output 2
Enter a number
-8
Sum is 0
The user provides a negative value in this field. Even if the test condition will be false, the loop’s function will still run once.
Infinite while loop
If a loop’s condition is always true, it will loop indefinitely (until the memory is full). For instance,
// infinite while loop
while(true){
// body of loop
}
Here is an example of an infinite do...while
loop.
// infinite do...while loop
int count = 1;
do {
// body of loop
} while(count == 1)
In the programmes mentioned above, text Expression is always true. As a result, the body of the loop will run indefinitely.
for and while loops
When the number of iterations is known, the for loop is utilised. For instance,
for (let i = 1; i <=5; ++i) {
// body of loop
}
When the number of iterations is uncertain, while and do…while loops are typically employed. For instance,
while (condition) {
// body of loop
}